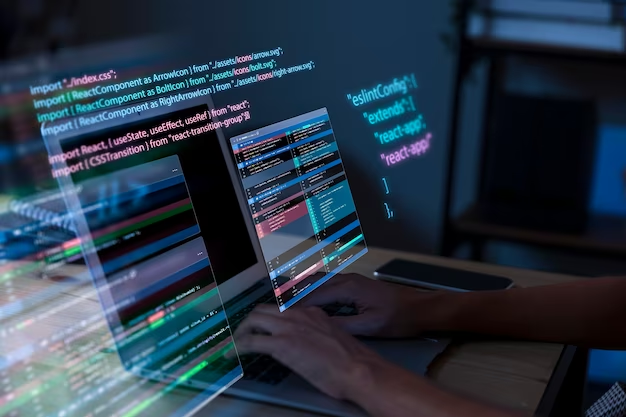
Understanding Java Fields and Methods
In the realm of Java programming, mastering the concepts of fields and methods is paramount. These fundamental building blocks empower developers to store, manipulate, and transform data, forming the backbone of robust applications.
In this tutorial, let’s delve into the concept of Java fields and methods and learn how to create and utilize them effectively.
Understanding Java Fields
Java classes are comprised of various fields and methods. Fields store specific data, while methods contain code that manipulates the data stored within these fields.
Java Fields: Storing Data
Fields are used for data storage and can be categorized into Primitive Types or Reference Types. Java offers 8 primitive types: int, byte, long, short, double, float, boolean, and char. Defining a field requires specifying the type, name, and value. For instance:
int num = 1;
If no value is assigned to a field, it assumes its default value. Default values for primitive types are as follows:
- int: 0;
- byte: 0;
- short: 0;
- long: 0L;
- float: 0.0f;
- double: 0.0d;
- char: ‘u0000’;
- boolean: false.
Reference types, such as String, are initially set to null.
Types of Fields
Java includes various types of fields:
- Local Variables: Defined within a method and accessible only within that method;
- Global Variables: Can be accessed by any method and are often declared at the top of the class;
- Parameters: Variables passed to methods upon invocation.
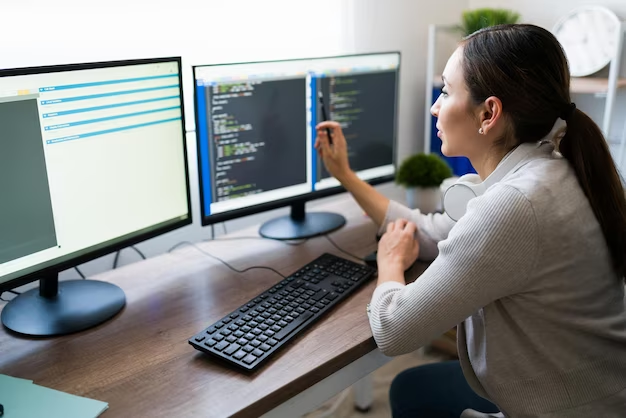
Java Methods: Manipulating Data
Methods are blocks of code responsible for manipulating field values and performing operations. To create a method, specify its name along with opening and closing parentheses and brackets. A basic method structure looks like this:
public void methodName() { // Code here }
Methods can also accept parameters for more flexibility:
public void methodName(int param1, int param2) { // Code here }
In the above example, param1 and param2 are the parameters passed to the method.
Calling Methods
Methods are called using their names. If a method doesn’t require parameters, simply use the method name followed by parentheses, like methodName(). If parameters are needed, specify their values within the parentheses, such as methodName(5, 3).
When dealing with methods from another class, use the syntax className.methodName().
Naming Conventions
Following naming conventions enhances code readability. Use camelCase for variables, fields, and methods. Additionally, start method names with verbs. For example, a method for adding numbers could be named addNum(). Field names should reflect real-world objects, aiding comprehension.
Example: Understanding Fields and Methods
Consider a class named Main containing global variables firstNum and secondNum, initialized as 5 and 10 respectively. A method addNum() is defined, and the main method calls it:
Main mains = new Main(); mains.addNum();
Inside addNum(), a local variable answer is initialized to 0. The code calculates the sum and prints the result. The output will be “The sum of two numbers is 15”.
In our next tutorial, we’ll delve into Java Access Levels.
Video Guide
To make it even clearer to you how to write the code, we picked up a video for you that explains it in detail.
Comparison of Java Fields and Methods
For your convenience, we have collected all the most common methods in one table, let’s pay attention to them.
Aspect | Java Fields | Java Methods |
---|---|---|
Purpose | Store data | Perform operations |
Data Types | Primitive or Reference | No direct data storage |
Accessibility | Local, Global, Parameters | Available based on access |
Initialization | Default or assigned value | Executed when called |
Invocation | Accessed directly | Called by name |
Naming Conventions | Reflect data, camelCase | Reflect action, camelCase |
Interaction with | Manipulated by methods | Operate on fields, data |
Conclusion
Mastering Java fields and methods is crucial for building powerful applications. These concepts serve as the foundation for data storage, manipulation, and execution of code operations.
FAQ
Java fields are variables that store data, while methods contain executable code to manipulate data or perform operations on it.
Fields serve as data holders in Java classes. They can store primitive types or reference types and provide the foundation for storing information.
Fields can be assigned initial values during declaration. If not assigned, they will assume default values based on their data types.
Java supports various types of fields, including local variables (limited to method scope), global variables (accessible across methods), and parameters (values passed to methods).