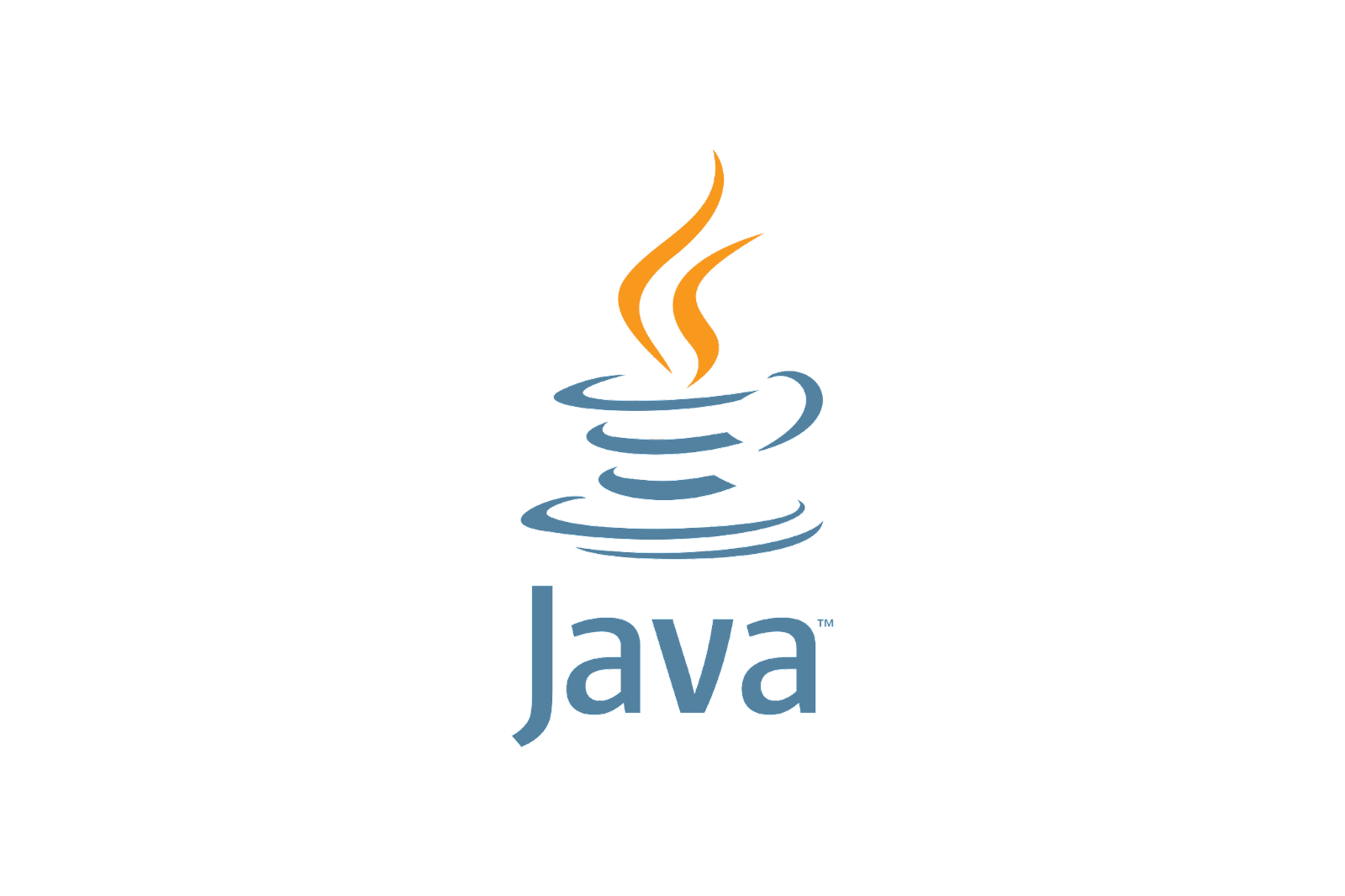
Terminating Loops In Java: Mastering Control Flow
Loops are fundamental constructs in programming that allow us to execute a block of code repeatedly. Java, as a versatile programming language, provides several methods to terminate loops based on specific conditions. Whether you’re a beginner or an experienced developer, understanding these termination techniques is crucial for writing efficient and maintainable code.
Using “break” Statement for Loop Termination
The Power of break
The break statement is a versatile tool in Java that enables you to exit a loop prematurely. This statement is especially handy when you want to terminate a loop based on a certain condition. Imagine you’re searching for a specific element in an array. Once you find it, using break can help you escape the loop, saving unnecessary iterations.
Example:
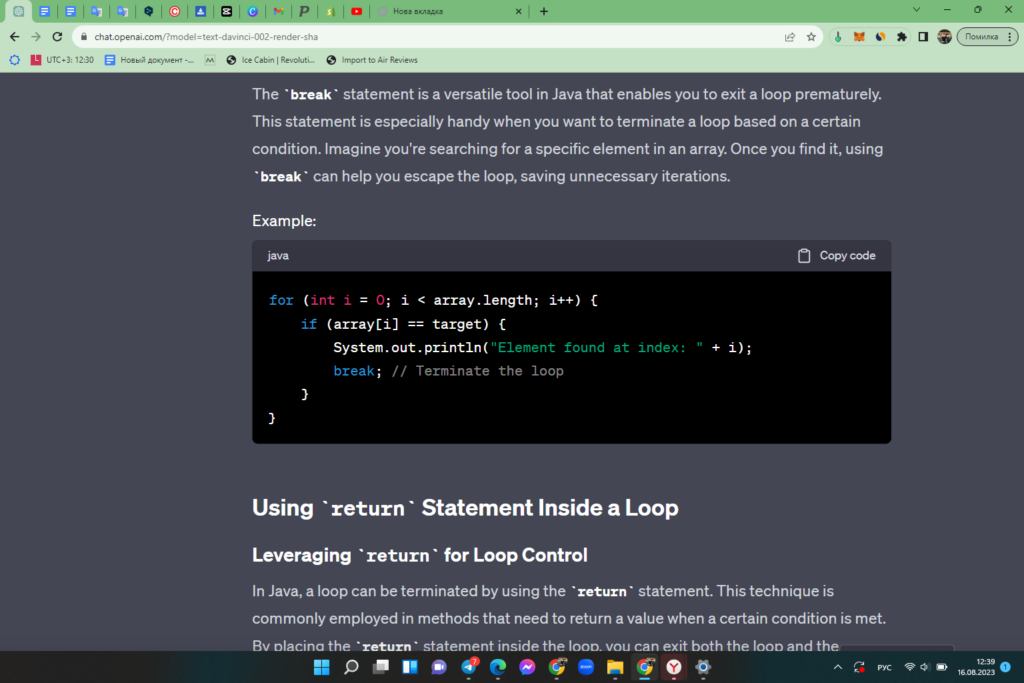
Using “return” Statement Inside a Loop
Leveraging return for Loop Control
In Java, a loop can be terminated by using the return statement. This technique is commonly employed in methods that need to return a value when a certain condition is met. By placing the return statement inside the loop, you can exit both the loop and the enclosing method simultaneously.
Example:
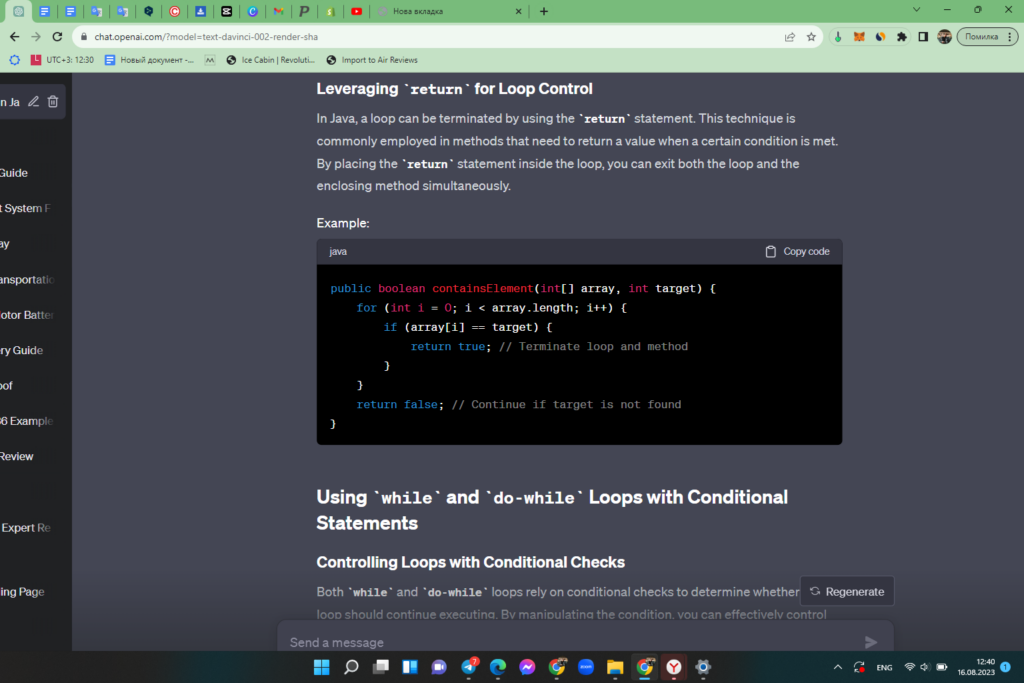
Using “while” and “do-while” Loops with Conditional Statements
Controlling Loops with Conditional Checks
Both while and do-while loops rely on conditional checks to determine whether the loop should continue executing. By manipulating the condition, you can effectively control when the loop terminates.
Example (while loop):
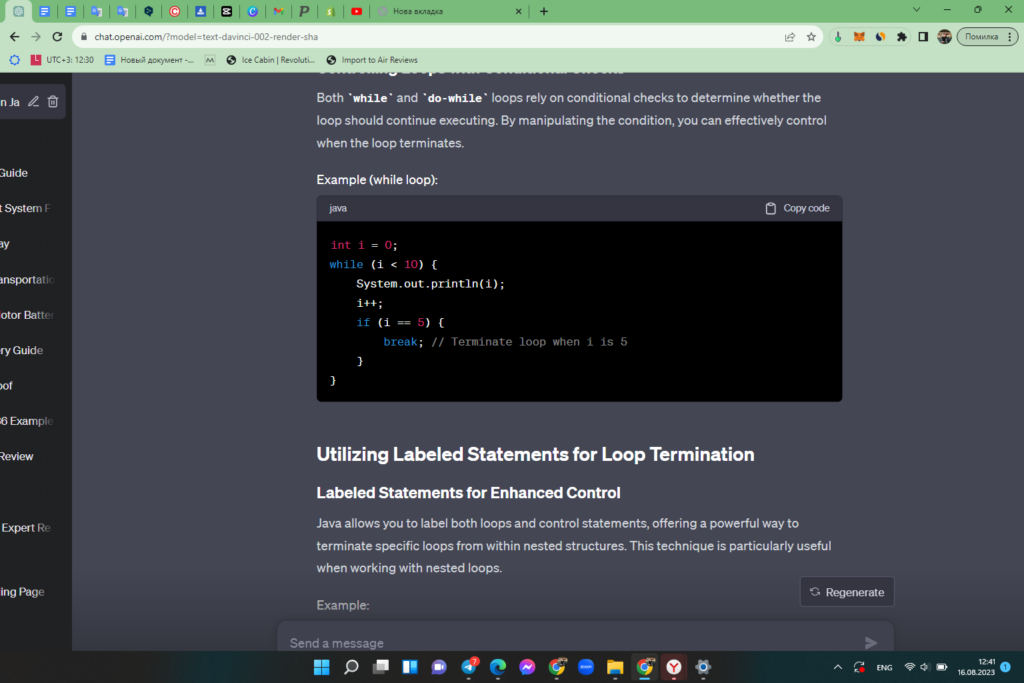
Utilizing Labeled Statements for Loop Termination
Labeled Statements for Enhanced Control
Java allows you to label both loops and control statements, offering a powerful way to terminate specific loops from within nested structures. This technique is particularly useful when working with nested loops.
Example:
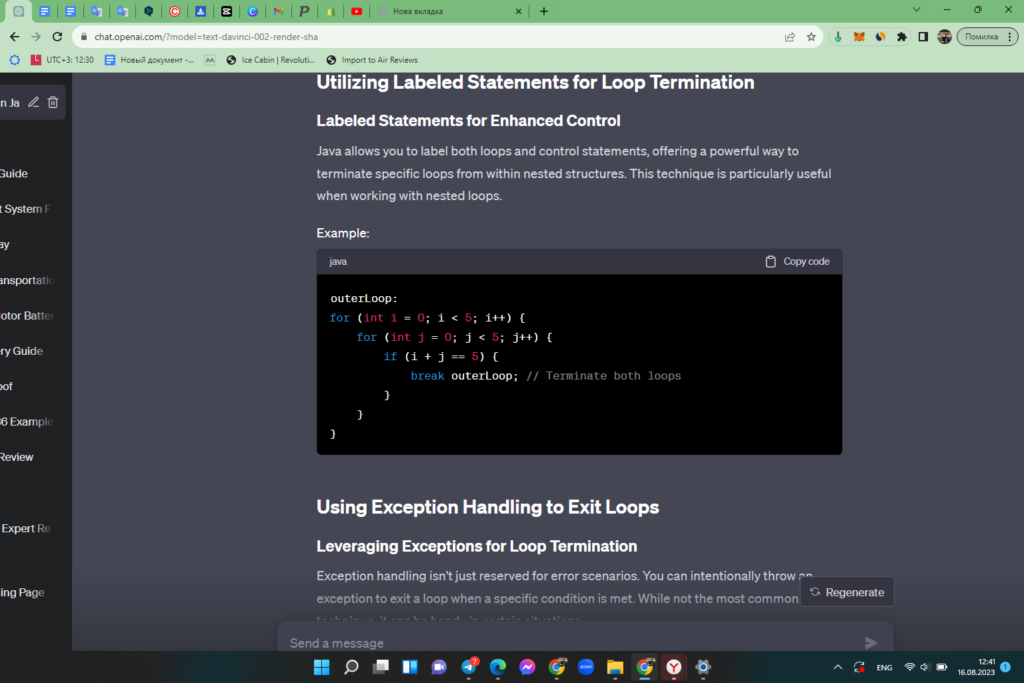
Using Exception Handling to Exit Loops
Leveraging Exceptions for Loop Termination
Exception handling isn’t just reserved for error scenarios. You can intentionally throw an exception to exit a loop when a specific condition is met. While not the most common technique, it can be handy in certain situations.
Example:
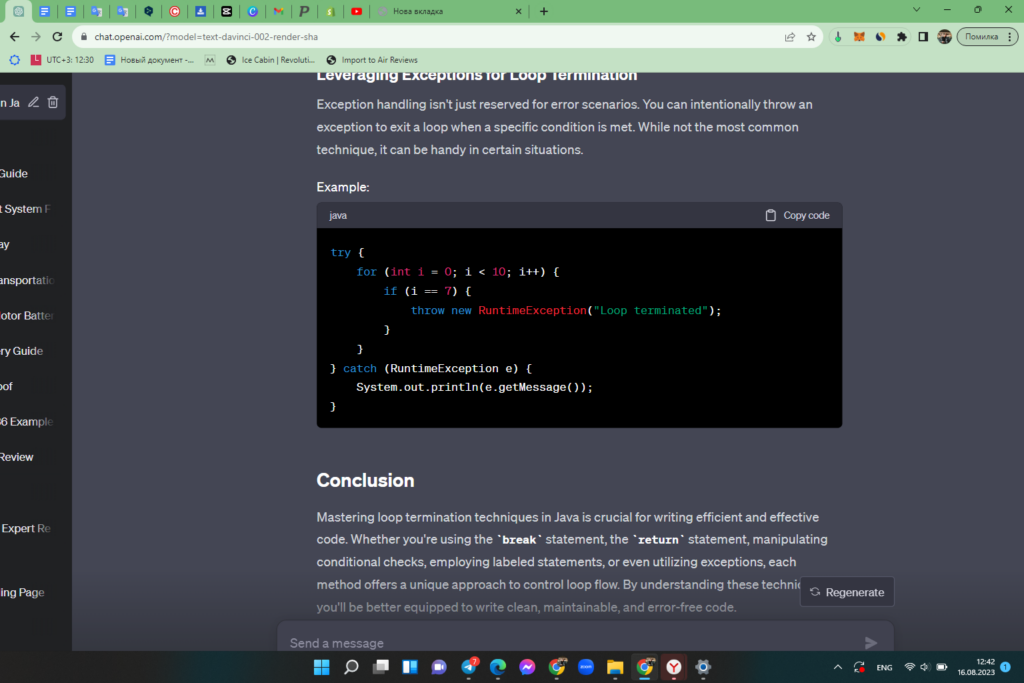
Utilizing “continue” Statement for Loop Skips
Skipping Iterations with continue
The continue statement is a powerful tool that allows you to skip the current iteration of a loop and proceed to the next one. This can be particularly useful when you want to exclude certain elements from processing within a loop.
Example:
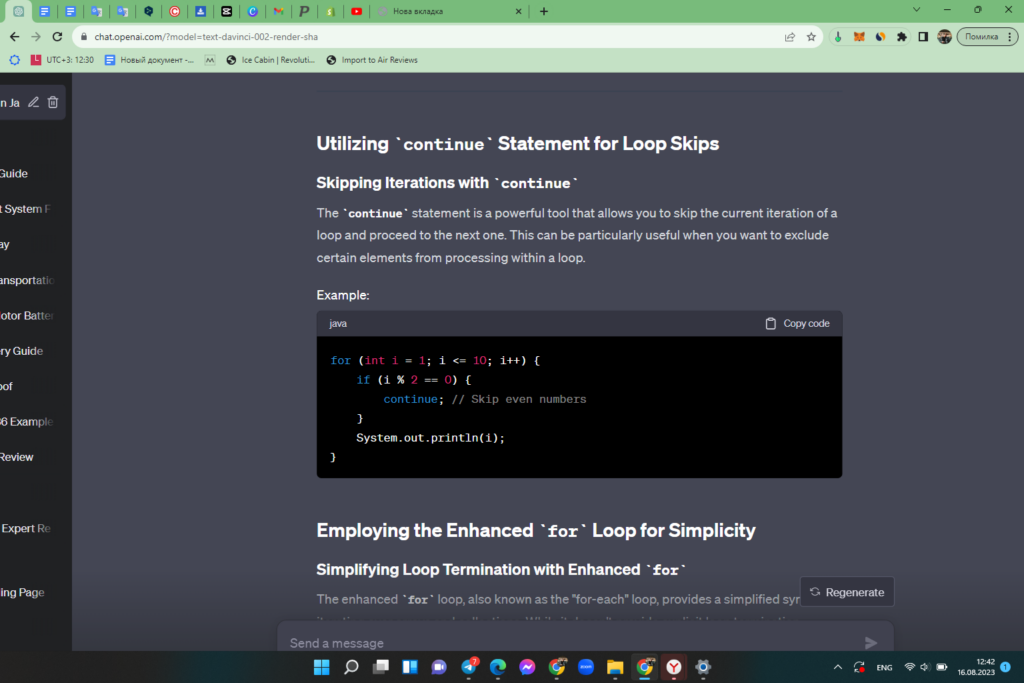
Employing the Enhanced “for” Loop for Simplicity
Simplifying Loop Termination with Enhanced “for”
The enhanced for loop, also known as the “for-each” loop, provides a simplified syntax for iterating over arrays and collections. While it doesn’t provide explicit loop termination mechanisms, its structure inherently terminates when all elements have been processed.
Example:
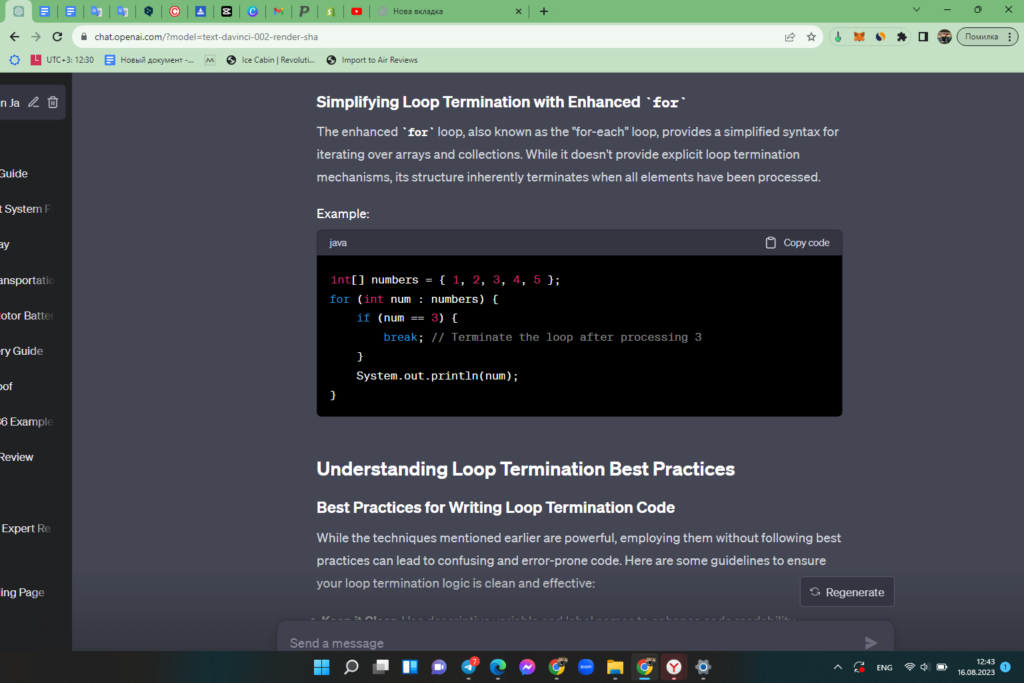
Exploring Infinite Loop Termination Strategies
Strategies to Escape Infinite Loops
In some cases, loops can unintentionally run indefinitely, causing your program to hang or crash. To mitigate this risk, it’s essential to have strategies in place to terminate infinite loops. Here are a couple of common techniques:
- Using a Counter: Implement a counter that keeps track of the number of iterations. Set a maximum threshold, and if the counter exceeds this threshold, break out of the loop. This ensures that even if the loop’s termination condition isn’t met, the loop will eventually stop.
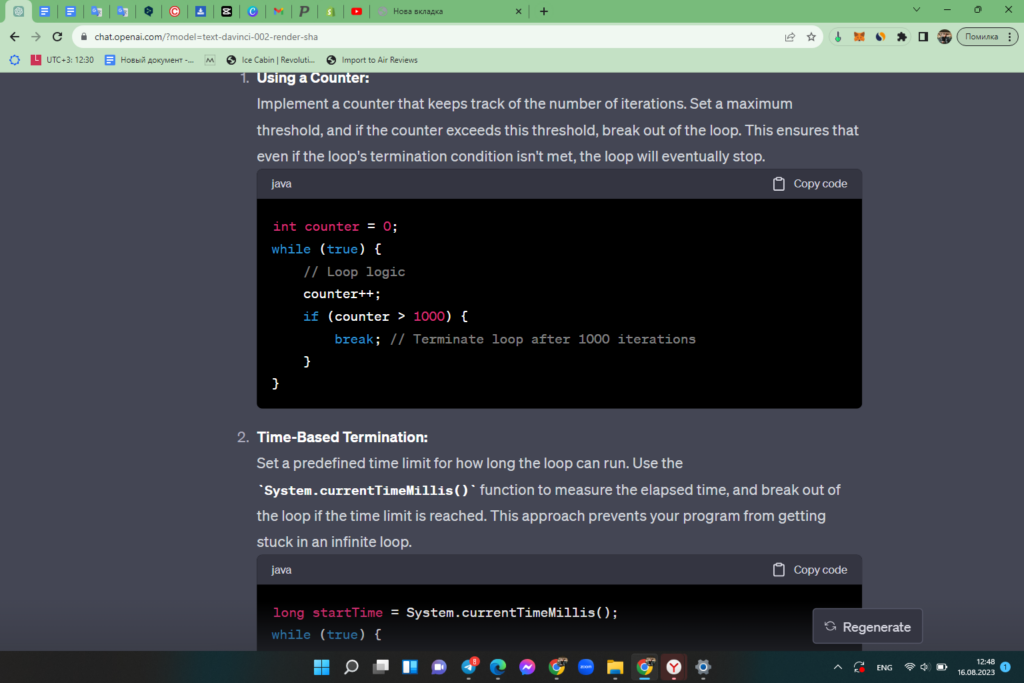
- Time-Based Termination: Set a predefined time limit for how long the loop can run. Use the System.currentTimeMillis() function to measure the elapsed time, and break out of the loop if the time limit is reached. This approach prevents your program from getting stuck in an infinite loop.
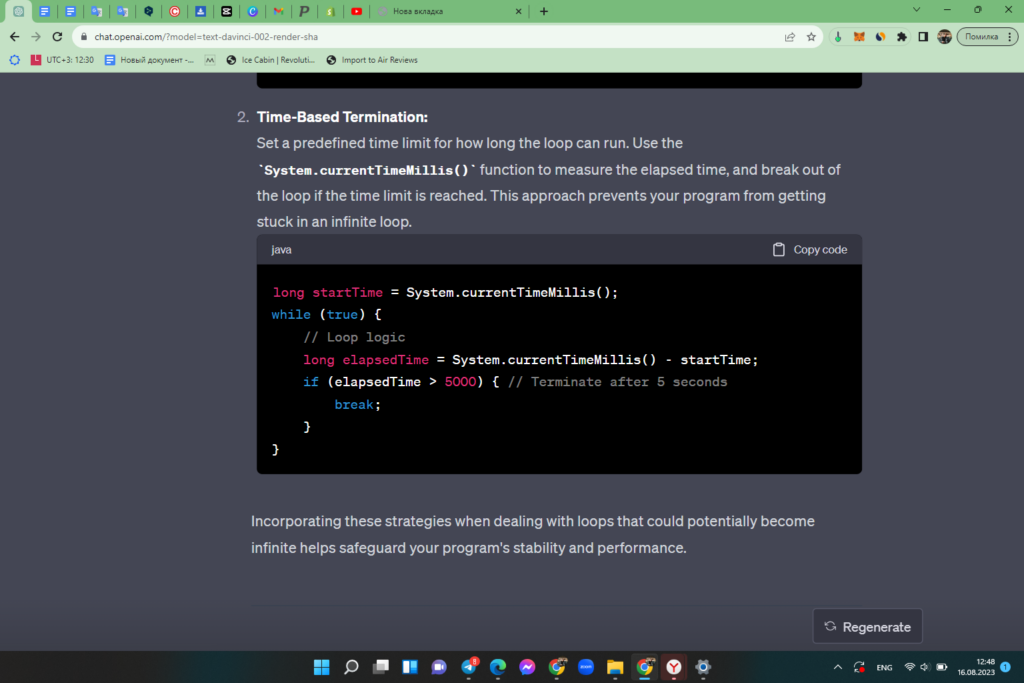
Understanding Loop Termination Best Practices
Best Practices for Writing Loop Termination Code
While the techniques mentioned earlier are powerful, employing them without following best practices can lead to confusing and error-prone code. Here are some guidelines to ensure your loop termination logic is clean and effective:
- Keep it Clear: Use descriptive variable and label names to enhance code readability;
- Minimize Nested Loops: Complex nested loops can make code hard to follow. Use labeled statements judiciously to control nested loop termination;
- Avoid Unintended Infinite Loops: Make sure your loop termination conditions are well-defined and tested;
- Use Break Sparingly: While break is useful, using it excessively can make code harder to understand. Consider refactoring your code if you find yourself using break extensively;
- Comment Your Intent: If you’re using less common techniques like labeled statements or exceptions, provide comments explaining their purpose and reasoning.
Conclusion
Mastering loop termination techniques in Java is crucial for writing efficient and effective code. Whether you’re using the break statement, the return statement, manipulating conditional checks, employing labeled statements, or even utilizing exceptions, each method offers a unique approach to control loop flow. By understanding these techniques, you’ll be better equipped to write clean, maintainable, and error-free code.
FAQs (Frequently Asked Questions)
Yes, you can use multiple break statements to terminate nested loops at different levels.
No, labeled statements can also be used for controlling other types of control structures, enhancing code readability.
Exceptions should be used judiciously for loop termination in situations where other techniques might not be suitable or clear.
No, the return statement not only terminates the loop but also exits the enclosing method, which may not be the case with break.
Labeled statements provide more fine-grained control over loop termination, especially in complex nested loop scenarios.