Java String Contains Char: Methods for Character Search
Java is a widely used, high-level programming language known for its versatility and portability. It was developed by Sun Microsystems (now owned by Oracle) and released in 1995. Java programs are compiled into an intermediate form called bytecode, which can run on any device with a Java Virtual Machine (JVM). This characteristic makes Java platform-independent, enabling developers to create applications that can run on different operating systems without modification. It’s commonly used for building web applications, mobile apps, desktop software, and more.
When working with strings in Java, it’s a common task to determine whether a particular character is present within the string. Whether you’re building a text processing application or working on a data validation task, the ability to check if a string contains a character is a fundamental skill. In this article, we’ll explore different methods to achieve this using Java.
Some examples
To determine whether a Java string includes a certain character, you can utilize the following techniques:
– The contains() method of the Java String class examines if a specific sequence of characters exists within the string. It yields a true result when the indicated character sequence is found within the string; otherwise, it produces a false outcome. The contains() method is case-sensitive.
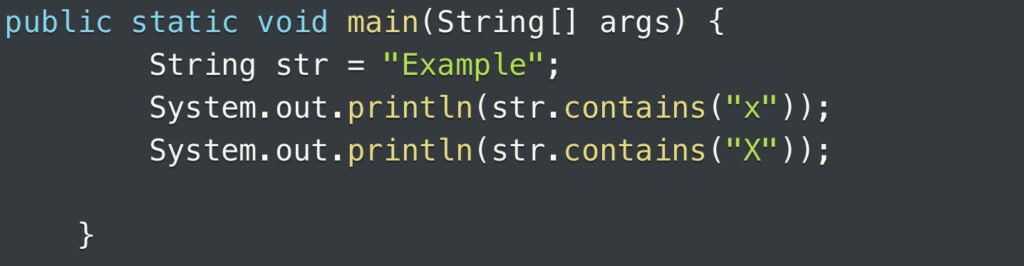
Output:
true
false
Through the utilization of the indexOf() method:
In contrast to the boolean output of the contains() method, the indexOf() method provides an integer result, representing the position of the substring or character within the string.
When fed with a String input, the indexOf() method delivers the initial position of the given string if found; otherwise, it delivers -1.
For instance, if you apply “Hello World”.indexOf(“World”), the result will be 6, whereas “Hello World”.indexOf(“world”) will yield -1.

Output:
The position of Tech is 9
By employing the charAt() method in combination with a loop:
Our approach involves iterating through the entire length of the string to identify a match for the specified character.
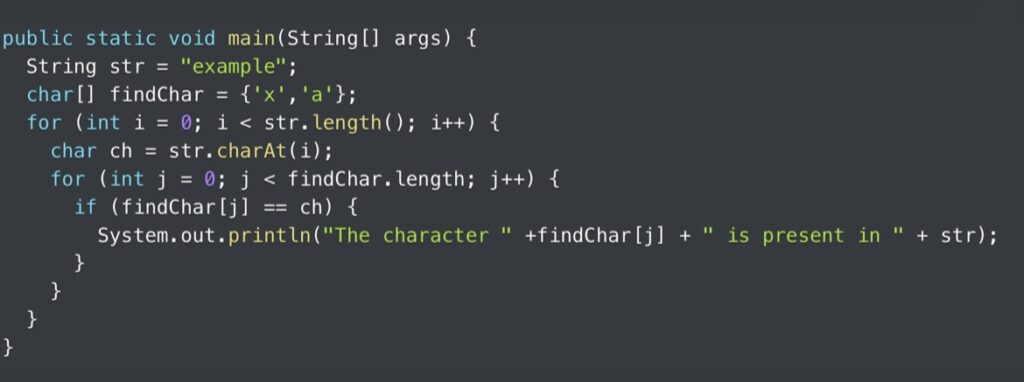
Output:
The character x is present in example
The character a is present in example
Using the Enhanced `for` Loop
Another approach to check if a string contains a character is by iterating through each character in the string using an enhanced `for` loop. This method is particularly useful if you need to perform additional actions when the character is found. Here’s an example:
“`java
String text = “OpenAI GPT-3.5”;
char targetChar = ‘A’;
boolean found = false;
for (char c : text.toCharArray()) {
if (c == targetChar) {
found = true;
break;
}
}
if (found) {
System.out.println(“The string contains the character.”);
} else {
System.out.println(“The string does not contain the character.”);
}
“`
In this code snippet, the enhanced `for` loop iterates through each character in the string “OpenAI GPT-3.5”. If the target character ‘A’ is found, the `found` flag is set to `true`, and the loop is terminated early.
Using Regular Expressions
For more complex scenarios, where you might need to match specific patterns, regular expressions can be a powerful tool. The `Pattern` class in Java provides methods for compiling and matching regular expressions. Here’s an example of how you can use regular expressions to check for the presence of a character:
“`java
import java.util.regex.*;
String text = “Regular expressions are versatile!”;
char targetChar = ‘v’;
Pattern pattern = Pattern.compile(String.valueOf(targetChar));
Matcher matcher = pattern.matcher(text);
if (matcher.find()) {
System.out.println(“The string contains the character.”);
} else {
System.out.println(“The string does not contain the character.”);
}
“`
In this code, a regular expression is created using the target character ‘v’. The `Matcher` class is then used to find a match within the input string. If a match is found, the output will indicate that the string contains the character.
Conclusion
Checking if a string contains a character in Java is a task that you’ll encounter frequently when working with text-based applications. Fortunately, Java provides various methods to accomplish this, ranging from simple approaches like using the `indexOf` and `contains` methods to more advanced techniques involving regular expressions. The choice of method depends on the complexity of your requirements and the additional processing you need to perform once the character is found.
The `indexOf` method returns the index of the first occurrence of a specified character in the string, or -1 if the character is not found. The `contains` method returns a boolean indicating whether the string contains the specified character or not.
For more complex scenarios, you can utilize regular expressions to search for patterns within the string. Regular expressions offer powerful tools for string matching and manipulation, enabling you to search for specific characters, patterns, or even groups of characters.