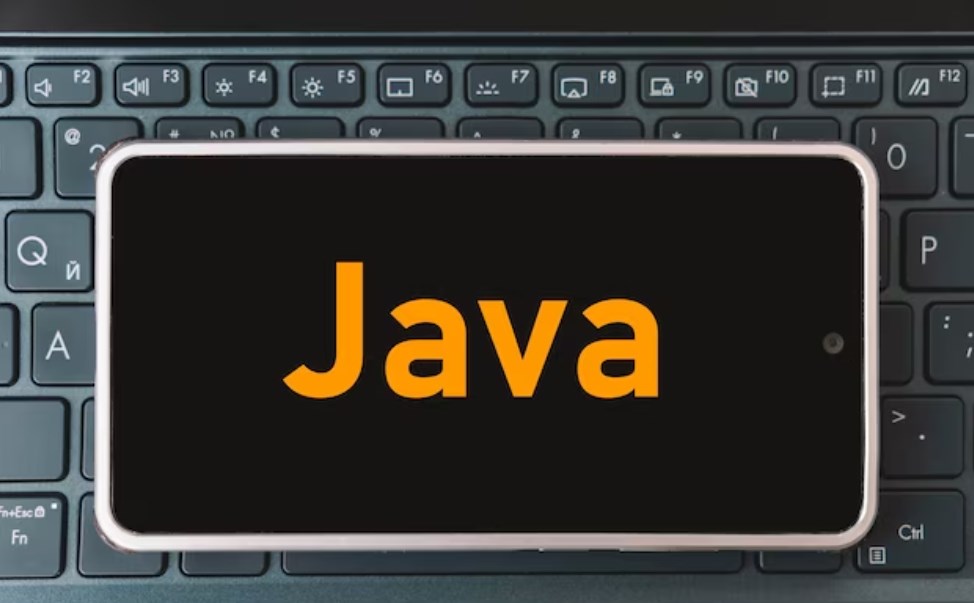
Mastering the Key Monitoring Technique in Java Applications
In the vast universe of the Java programming language, capturing and responding to user keyboard input remains a fundamental skill. Leveraging the capabilities of KeyListener offers developers a direct window into user actions, enabling dynamic interactions and intuitive interfaces.
Whether you’re looking to create an immersive game or a responsive software tool, mastering the art of key monitoring is pivotal. This article unfolds a comprehensive guide to embedding this functionality within your Java projects.
The Essence of Key Monitoring
KeyListener, or what some developers prefer to call “key event watcher,” is a prominent interface present within the Java AWT (Abstract Window Toolkit) library. Its primary role is to detect and respond to keyboard actions. Essentially, when a user presses, releases, or types a key, the key event watcher is ready to act, letting software respond in kind.
<iframe width=”560″ height=”315″ src=”https://www.youtube.com/embed/BJ7fr9XwS2o” title=”YouTube video player” frameborder=”0″ allow=”accelerometer; autoplay; clipboard-write; encrypted-media; gyroscope; picture-in-picture; web-share” allowfullscreen></iframe>
1. Understanding the Interface
Before diving into practical programming insights, it’s crucial to understand the three main methods the interface offers:
- keyTyped(KeyEvent e): This function is called when a key is typed (i.e., pressed and released);
- keyPressed(KeyEvent e): Invoked when a key is pressed down;
- keyReleased(KeyEvent e): Called when a key is let go.
Each of these functions receives a KeyEvent object which contains information about the key action, allowing developers to design specific responses.
2. Setting the Groundwork
To harness the power of the key event watcher, it’s necessary to include specific libraries:
java
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
Next, the class that requires this functionality should implement the interface. Let’s create a simple JFrame that listens to key events.
java
import javax.swing.JFrame;
public class KeyMonitoringExample extends JFrame implements KeyListener {
public KeyMonitoringExample() {
this.setTitle(“KeyListener Demonstration”);
this.setSize(400, 200);
this.addKeyListener(this);
this.setVisible(true);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
// The key event watcher methods go here
public static void main(String[] args) {
new KeyMonitoringExample();
}
}
3. Embedding Key Event Watcher Functions
With the foundation set, integrate the key watcher methods:
java
@Override
public void keyTyped(KeyEvent e) {
System.out.println(“Key typed: ” + e.getKeyChar());
}
@Override
public void keyPressed(KeyEvent e) {
System.out.println(“Key pressed: ” + e.getKeyCode());
}
@Override
public void keyReleased(KeyEvent e) {
System.out.println(“Key released: ” + e.getKeyCode());
}
4. Practical Usage Scenarios:
- Gaming: If developing an interactive game, key monitoring can be essential. For instance, the WASD keys could control a character’s movement;
- Shortcut Implementation: Modern software solutions often utilize keyboard shortcuts for functions like copy, paste, save, etc. Monitoring key events makes this possible;
- Interactive Tutorials: Detecting key presses can guide users through software tutorials, responding to their actions in real time.
5. Tips and Tricks:
- Debounce: Sometimes, it’s useful to ignore repeated key presses in a short span. This can prevent unintended rapid actions;
- Combination Detection: Detecting multiple key presses at once (e.g., CTRL + C) can be achieved by checking the status of multiple keys within the keyPressed and keyReleased methods.
Beyond the Basics: Advanced Key Event Handling
The core knowledge of key event watching is just the starting point. Let’s dive deeper into the ocean of Java key event capabilities to ensure you are fully equipped to address any challenges or demands that arise in the dynamic environment of user interactions.
Character vs Keycode
When working with key events, understanding the distinction between character and keycode becomes imperative:
- Character: Represented by getKeyChar(), it fetches the Unicode character associated with the key. This is particularly useful for typing-sensitive applications or text editors;
- Keycode: Retrieved using getKeyCode(), it indicates the physical key pressed, devoid of its character representation. Ideal for gaming or software requiring specific key commands, irrespective of the character it represents.
Modifier Keys and Their Power
Modifier keys such as Shift, Ctrl, and Alt act as agents that change the original function of another key when pressed simultaneously. Java’s key event framework provides the ability to detect these using methods like isShiftDown(), isControlDown(), and isAltDown(). This opens up a new dimension for complex command execution within your application.
Key Bindings: An Alternative to KeyListeners
In certain situations, Key Bindings may be a preferred approach over key event watchers. Key bindings allow the action to be associated directly with a key stroke, bypassing the need for low-level key event handling. It offers a more robust solution, especially when working with Swing components.
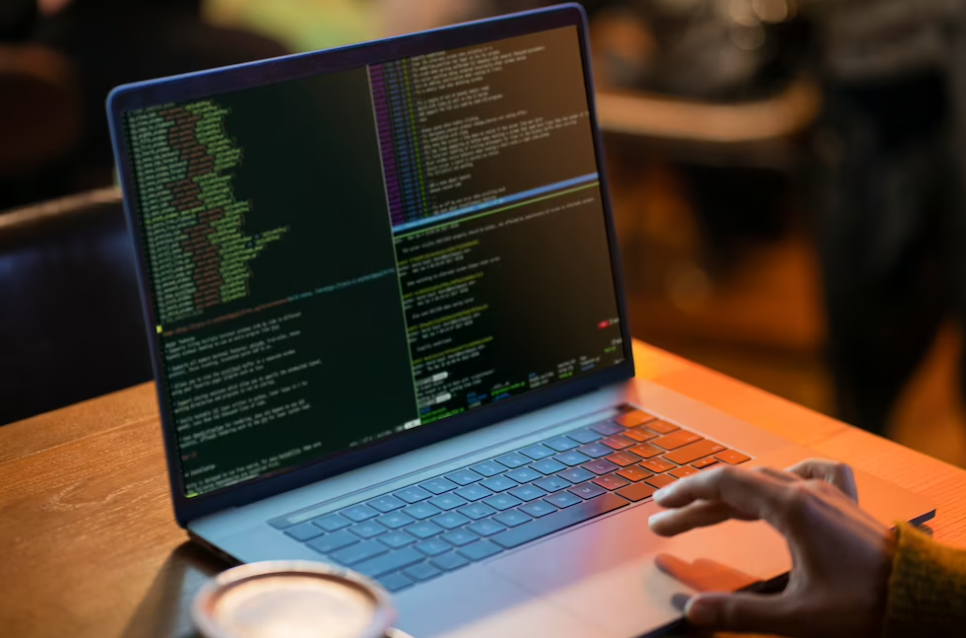
Filter Specific Key Events
There might be situations where you only wish to detect a certain category of keys – alphabets, numbers, or function keys. Instead of a generic approach, the KeyEvent class provides constants (e.g., KeyEvent.VK_A for the ‘A’ key) that allow for specific key event handling.
Debounce Mechanism in Depth
The concept of debouncing was touched upon earlier, but it’s worth diving deeper. In applications where rapid key presses can lead to glitches or unintentional behavior, a debounce mechanism can be a lifesaver. By setting up a small delay after a key event is detected, you can ensure that additional events from the same key within this delay are ignored.
Key Event Handling in Modern Java Frameworks
While AWT offers a foundational approach, modern frameworks like JavaFX provide enriched event handling capabilities. For instance, JavaFX supports multi-touch points, making it suitable for devices that allow multiple simultaneous key presses.
Handling International Keyboard Layouts
For applications targeting a global audience, considering different keyboard layouts is crucial. Unicode character representation becomes essential here, ensuring that regardless of the physical key position, the character input remains consistent across various layouts.
Performance Considerations
When crafting highly responsive applications, the efficiency of your key event handling logic can have a pronounced impact. Avoid heavy computations or I/O operations within the key event methods. Instead, consider queuing such operations or offloading them to separate threads to maintain a smooth user experience.
Common Pitfalls
Swing’s Event Dispatch Thread (EDT): When working with Swing, remember that key event handlers run on the EDT. Any long-running operation can cause the UI to freeze.
Simultaneous Key Presses: Not all keyboards support n-key rollover (the ability to recognize multiple keys pressed simultaneously). It’s a good practice to test applications on different hardware.
Resources for Further Learning
Java offers extensive documentation on its key event functionalities. Apart from the official documentation, numerous forums, online courses, and books provide in-depth insights into advanced key event handling techniques.
Conclusion
The art of integrating key event watching in Java is more than just embedding a few methods. It’s about understanding user interactions, foreseeing their needs, and crafting fluid, intuitive responses. While the AWT library offers a robust foundation, mastering this art will inevitably uplift your Java application to a pinnacle of user experience. Now, equipped with these insights, you’re primed to weave key monitoring seamlessly into your projects.