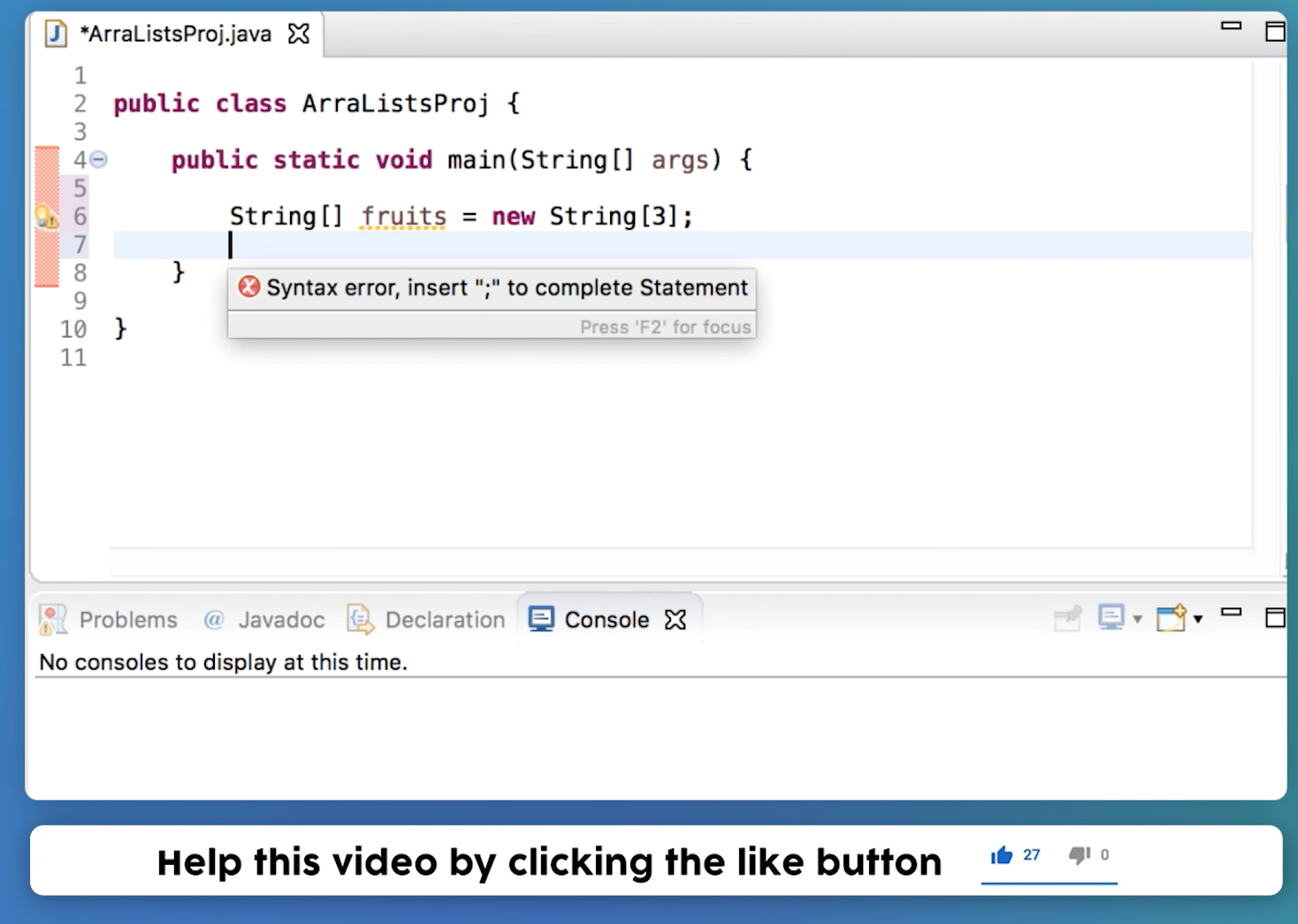
How to Print an ArrayList in Java
Java is a versatile and widely-used programming language that provides a plethora of tools and libraries to make developers’ lives easier. One such tool is the `ArrayList`, which is a part of the Java Collections Framework and provides a dynamic array-like structure to store and manipulate elements. In this article, we will delve into the techniques and methods for printing an `ArrayList` in Java, along with illustrative examples to help you understand the concepts better.
Introduction to ArrayList in Java
Before we jump into printing an ArrayList, let’s briefly review what an `ArrayList` is in Java. An `ArrayList` is a resizable array that is part of the Java Collections Framework. It provides dynamic storage, which means it can grow or shrink as needed to accommodate elements. This makes it a popular choice when you need to work with a dynamic collection of objects.
To use `ArrayList`, you need to import it using the following statement:
import java.util.ArrayList;
Printing ArrayList Elements
Printing the elements of an `ArrayList` is a common task when you want to display the content of the list to the user or for debugging purposes. There are several ways to achieve this.
Printing ArrayList using a print statement
Printing the contents of an ArrayList in Java can be accomplished through a straightforward approach using a simple print statement. This method is particularly useful when a quick overview of the ArrayList’s elements is needed, making it a handy tool for debugging and validation purposes.
Here’s an example that illustrates how to print an ArrayList using a print statement:
import java.util.ArrayList;
public class ArrayListPrintingExample {
public static void main(String[] args) {
ArrayList<Integer> numbers = new ArrayList<>();
numbers.add(10);
numbers.add(20);
numbers.add(30);
System.out.println("ArrayList contents: " + numbers);
}
}
In this instance, we start by importing the required packages. An ArrayList named `numbers` is populated with three integer values. To print the contents of the ArrayList, we utilize the `System.out.println()` statement. By concatenating the ArrayList object directly within the print statement, Java’s automatic string conversion comes into play, displaying the entire contents of the ArrayList within the printed string. This results in an output that clearly shows the values stored in the ArrayList.
While this approach offers a convenient way to quickly inspect the contents of an ArrayList, it may not provide the same level of control and formatting as more specialized printing techniques. However, for situations where a concise representation of the ArrayList’s data is sufficient, using a print statement is an efficient choice.
Using a Loop
One of the most straightforward methods is to use a loop, such as the `for` loop or the enhanced `for-each` loop, to iterate through the `ArrayList` and print each element individually.
ArrayList<String> fruits = new ArrayList<>();
fruits.add("Apple");
fruits.add("Banana");
fruits.add("Orange");
for (String fruit : fruits) {
System.out.println(fruit);
}
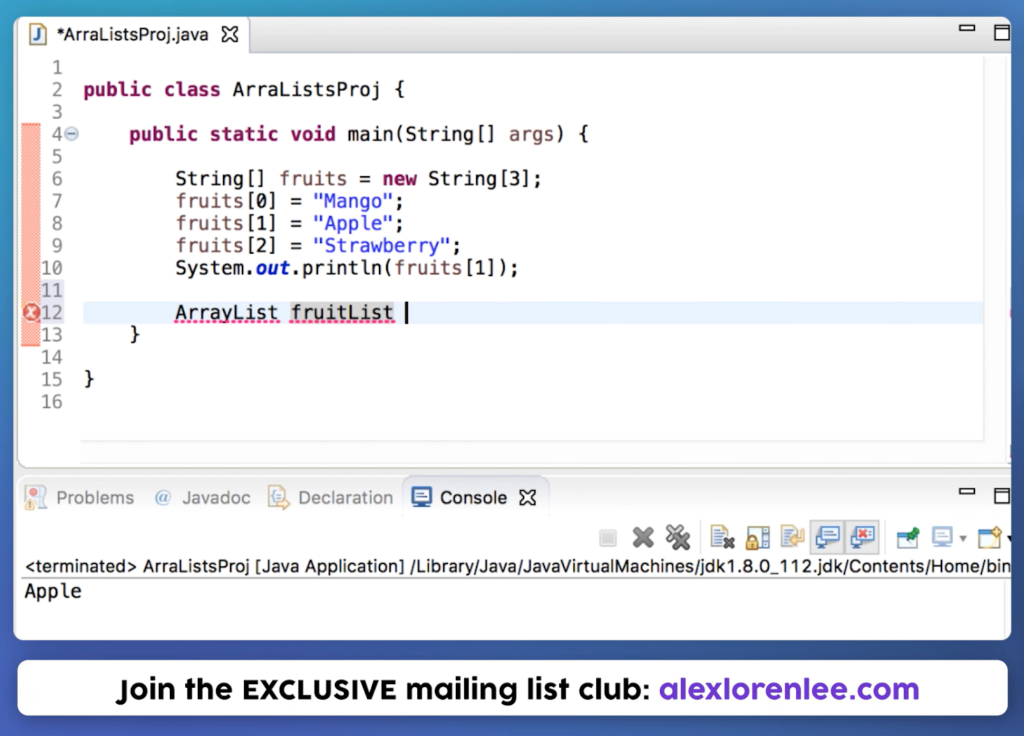
Using the toString() Method
The `ArrayList` class overrides the `toString()` method to provide a string representation of its content. This means you can directly use the `System.out.println()` method to print the entire `ArrayList`.
ArrayList<Integer> numbers = new ArrayList<>();
numbers.add(10);
numbers.add(20);
numbers.add(30);
System.out.println(numbers);
Using Java 8 Streams
With the introduction of Java 8, you can utilize the powerful Streams API to print the elements of an `ArrayList`.
ArrayList<String> colors = new ArrayList<>();
colors.add("Red");
colors.add("Green");
colors.add("Blue");
colors.stream().forEach(System.out::println);
Printing ArrayList using Iterator/ListIterator
Printing an ArrayList in Java is a fundamental task when it comes to working with collections of data. Among the various techniques available, utilizing an Iterator or a ListIterator provides a systematic and efficient approach to achieve this goal. Both Iterator and ListIterator are interfaces that allow for the traversal of elements in a collection while providing methods to access and manipulate the data. In this context, the `hasNext()` method checks for the presence of the next element, while the `next()` method retrieves and moves the iterator to the next element in the sequence.
Consider the following example, which demonstrates the process of printing an ArrayList using an Iterator:
import java.util.ArrayList;
import java.util.Iterator;
public class ArrayListPrintingExample {
public static void main(String[] args) {
ArrayList<String> fruits = new ArrayList<>();
fruits.add("Apple");
fruits.add("Banana");
fruits.add("Orange");
Iterator<String> iterator = fruits.iterator();
while (iterator.hasNext()) {
String fruit = iterator.next();
System.out.println(fruit);
}
}
}
In this illustration, we begin by importing the necessary packages. An ArrayList named `fruits` is populated with three different fruit names. To traverse and print the elements of this ArrayList, we obtain an Iterator using the `iterator()` method provided by the ArrayList class. The `while` loop is then utilized to iterate through the collection. Within each iteration, the `hasNext()` method is used to check if there are more elements left to traverse. If an element is present, the `next()` method is invoked to retrieve and subsequently print the element. This process continues until all elements have been printed.
The beauty of using an Iterator lies in its simplicity and efficiency. It provides a straightforward way to access the elements of a collection sequentially, abstracting away the internal implementation details of the ArrayList. Moreover, Iterators are applicable to a wide range of collection types, making the code adaptable to various scenarios.
While Iterators offer unidirectional traversal, Java also provides the ListIterator interface, which extends the capabilities of Iterator by enabling bidirectional traversal. However, for the purpose of clarity, the example focused on the basic Iterator usage. The approach showcased in the example is a cornerstone technique in Java programming, aiding developers in effectively managing and displaying the contents of ArrayLists.
Formatting Output
When printing an `ArrayList`, you might want to format the output to make it more visually appealing. You can use loops or streams in combination with formatting techniques to achieve this.
ArrayList<Double> prices = new ArrayList<>();
prices.add(9.99);
prices.add(19.95);
prices.add(5.49);
for (Double price : prices) {
System.out.printf("Price: $%.2f%n", price);
}
Handling Edge Cases
It’s important to consider edge cases while printing an `ArrayList`. What if the list is empty? To handle such scenarios gracefully, you can add a conditional check before printing.
ArrayList<String> books = new ArrayList<>();
if (books.isEmpty()) {
System.out.println("The list of books is empty.");
} else {
for (String book : books) {
System.out.println(book);
}
}
Conclusion
Printing the content of an `ArrayList` is a fundamental task in Java programming. By understanding the different approaches and methods available, you can choose the one that best suits your requirements. Whether you opt for loops, the `toString()` method, or Java 8 Streams, the ability to effectively print `ArrayList` elements will undoubtedly enhance your programming skills and enable you to create more informative and user-friendly applications.