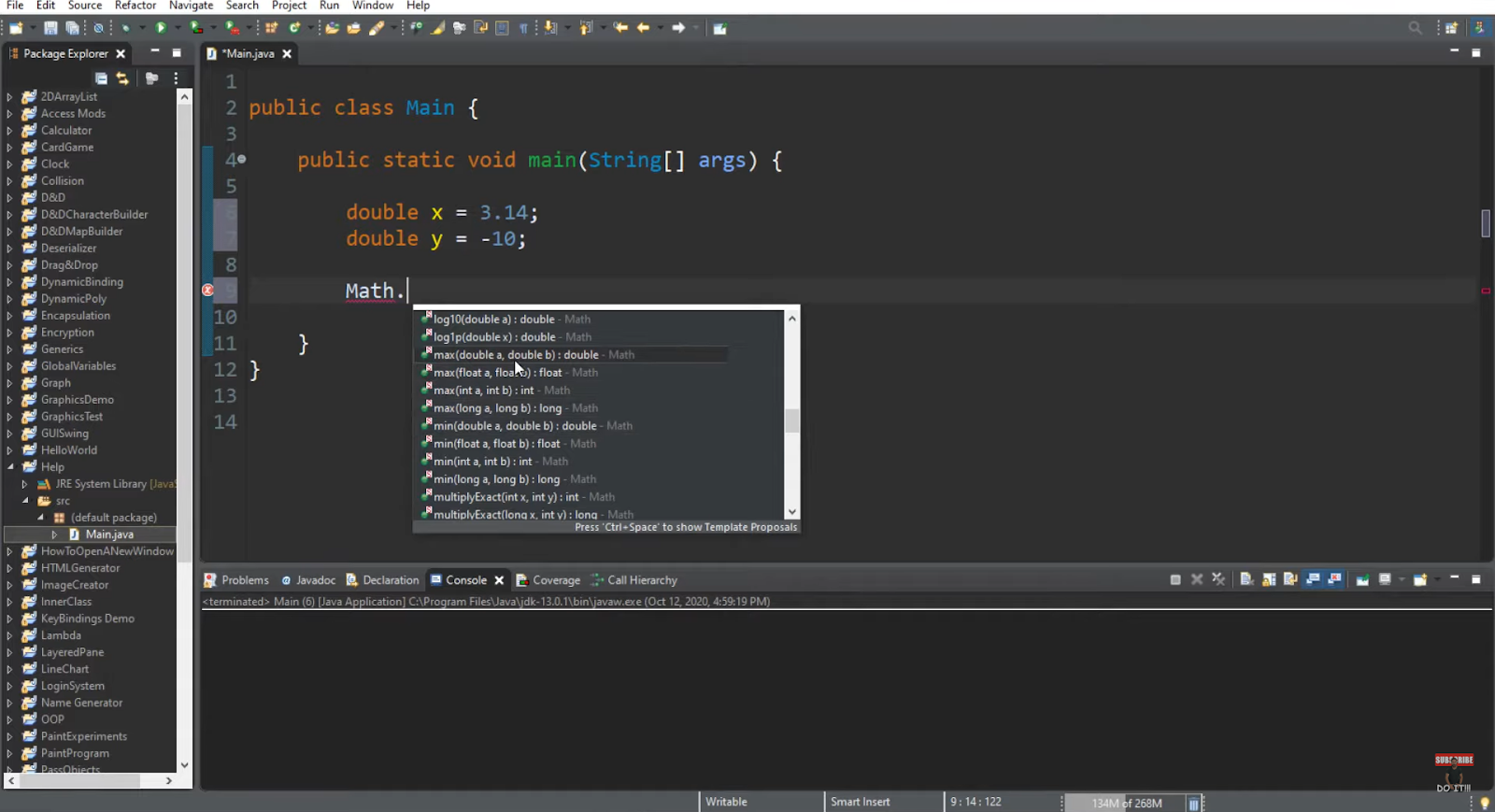
How to Import Math in Java: A Guide
In the Java programming language, the Math class is part of the java.lang package. This package serves as the default package for all programs, eliminating the necessity to import the Math class explicitly. However, it’s important to note that all the elements within the Math class, including both methods and variables, are of a static nature.
Accessing the static variables or methods provided by the Math class can be achieved through two distinct approaches.
Importing the Math Library: An Overview
Java’s Math library resides in the `java.lang` package, which is automatically available in every Java program. This means that you don’t need to explicitly import the Math class to utilize its functions. The Math class comprises various static methods and constants that facilitate tasks ranging from basic arithmetic operations to advanced trigonometry and logarithmic calculations.
Importing Math Functions: Examples
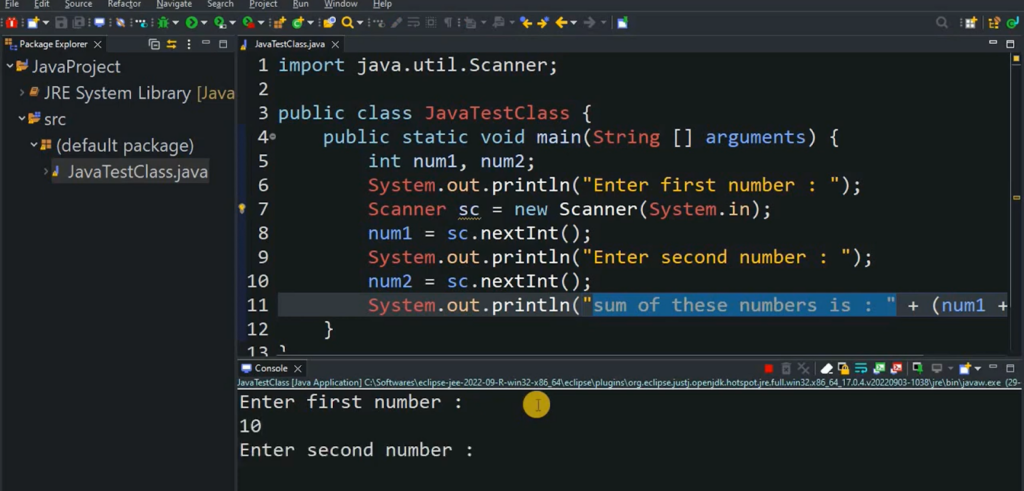
Basic Arithmetic Operations:
int sum = Math.addExact(5, 3); // Adds two numbers and handles overflow
int difference = Math.subtractExact(10, 4); // Subtracts two numbers and handles overflow
int product = Math.multiplyExact(6, 7); // Multiplies two numbers and handles overflow
Exponential and Logarithmic Functions:
double power = Math.pow(2, 3); // Calculates 2 raised to the power of 3
double logarithm = Math.log(10); // Calculates the natural logarithm of 10
Trigonometric Functions:
double sine = Math.sin(Math.toRadians(30)); // Calculates sine of 30 degrees
double cosine = Math.cos(Math.toRadians(45)); // Calculates cosine of 45 degrees
Rounding and Absolute Values:
int roundedValue = Math.round(6.8f); // Rounds 6.8 to the nearest integer
double absoluteValue = Math.abs(-9.5); // Calculates the absolute value of -9.5
Accessing Constants
Java’s Math class also provides useful constants:
double pi = Math.PI; // Retrieves the value of pi (3.141592653589793)
double euler = Math.E; // Retrieves the value of Euler's number (2.718281828459045)
Tips for Effective Math Imports
When working with Java programming, it’s important to optimize your code by avoiding redundant imports. An illustrative case is the Math class, nestled within the `java.lang` package, which eliminates the need for explicit imports. Importing it again would only lead to redundancy, so it’s best to steer clear of this practice. Moreover, the Math class exclusively harbors static methods, meaning they’re accessible directly through the class name itself, such as `Math.methodName()`. This approach underscores the nature of these methods, streamlining their invocation. Additionally, in scenarios where trigonometric functions demand angles in radians, remember to capitalize on the `Math.toRadians()` function. By using this method, you can effortlessly convert degrees to radians, ensuring the seamless integration of these functionalities into your code.
Topic | Description |
---|---|
Avoid Redundant Imports | Since the Math class is in the java.lang package, you don’t need to import it explicitly. Attempting to do so will result in redundancy. |
Static Access | All the methods in the Math class are static. Access them using the class name directly, like Math.methodName(). |
Type Conversion | Some methods (e.g., trigonometric functions) require angle inputs in radians. Use Math.toRadians() to convert degrees to radians. |
Accessing static variables and methods through the class name
In Java, static variables and methods within a class can be accessed directly using the class name, followed by a dot and the name of the static variable or method. This is particularly useful when dealing with Java’s Math class, which houses a plethora of static mathematical functions and constants. For example, if you want to calculate the square root of a number, you can access the `sqrt` method of the Math class like this:
double number = 25.0;
double squareRoot = Math.sqrt(number);
System.out.println("Square root of " + number + " is " + squareRoot);
In this example, the static method `sqrt` from the Math class is accessed using the class name `Math`, followed by the method name `sqrt()`. This direct access simplifies the utilization of static methods and variables without requiring an instance of the class.
Accessing static variables and methods by statically importing Math class
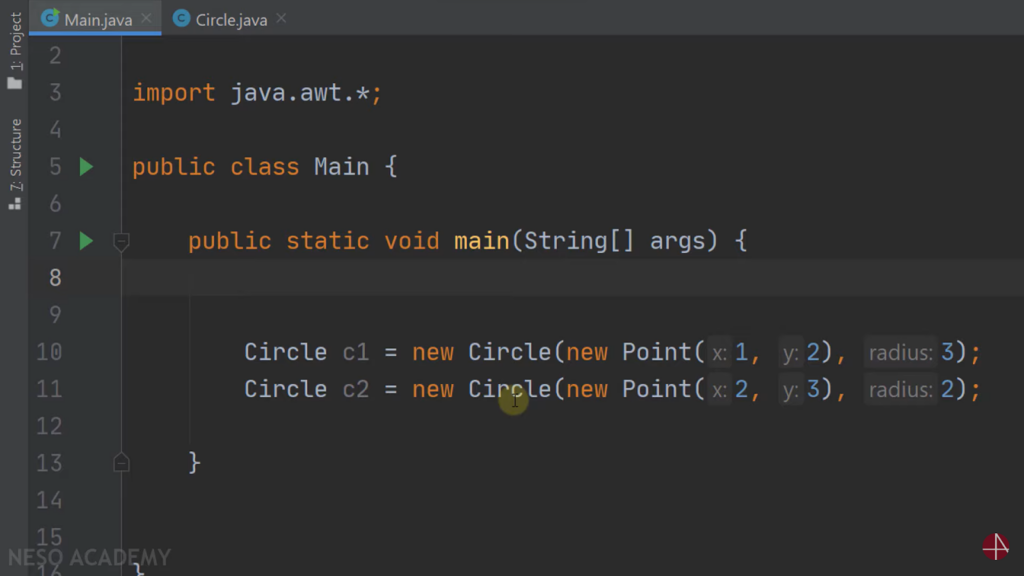
Java allows you to improve code readability and simplify access to static methods and variables by using static imports. By statically importing the Math class, you can directly use its static members without having to prefix them with the class name. This can make your code more concise and intuitive. Here’s how you can statically import the Math class and use it to calculate the sine of an angle:
import static java.lang.Math.*;
public class TrigonometryExample {
public static void main(String[] args) {
double angle = 45.0;
double sineValue = sin(toRadians(angle));
System.out.println("Sine of " + angle + " degrees is " + sineValue);
}
}
In this code, the `import static java.lang.Math.*`; statement enables you to directly use the static methods and constants from the Math class, like `sin()` and `toRadians()`, without the need to prepend `Math.` before each usage. This enhances code readability and makes your intentions clear, especially when working extensively with a particular class like Math.
Conclusion
In this article, we’ve explored the process of importing math in Java, showcasing the versatility of the Math library through code examples. By tapping into Java’s built-in mathematical functions, you can significantly enhance your coding efficiency and simplify intricate calculations. Whether you’re dealing with basic arithmetic, complex exponentials, or trigonometric operations, Java’s Math library equips you with a powerful toolset to conquer various mathematical challenges with ease.