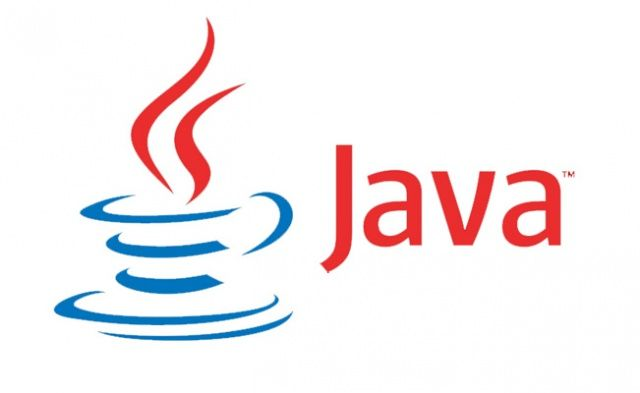
How to Implement MouseListener In Java: Step-by-Step Guide
Java programming offers an array of tools and functionalities for creating dynamic applications, and handling user interactions is a crucial aspect of it. One essential task is implementing the MouseListener interface, allowing your program to respond to mouse events. Whether you’re a beginner or an experienced developer, this guide will walk you through the process step by step, providing practical examples, code snippets, and best practices.
Understanding MouseListener Basics
Before diving into implementation, let’s grasp the fundamental concepts:
What is MouseListener?
MouseListener is an interface in Java’s java.awt.event package that provides methods to handle various mouse events. These events include clicking, pressing, releasing, entering, and exiting mouse components.
Why MouseListener Matters
MouseListener is crucial for applications that require user interaction, such as games, graphical editors, and UI-driven programs. It enables you to create responsive and user-friendly interfaces.
Setting Up Your Environment
To start implementing MouseListener, ensure you have:
- Java Development Kit (JDK): Download and install the latest JDK from the official Oracle website;
- Integrated Development Environment (IDE): Choose an IDE like Eclipse, IntelliJ IDEA, or NetBeans for efficient coding.
Implementing MouseListener Interface
Follow these steps to implement MouseListener in your Java program:
Step 1: Import Required Packages

Step 2: Implement the MouseListener Interface
Create a class that implements the MouseListener interface:
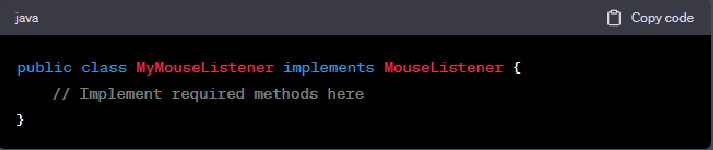
Step 3: Override MouseListener Methods
In your class, override the methods from the MouseListener interface:
- mouseClicked(MouseEvent e): Triggered when a mouse button is clicked;
- mousePressed(MouseEvent e): Invoked when a mouse button is pressed;
- mouseReleased(MouseEvent e): Called when a mouse button is released;
- mouseEntered(MouseEvent e): Activated when the mouse enters a component;
- mouseExited(MouseEvent e): Fired when the mouse exits a component.
Here’s an example of the implementation:
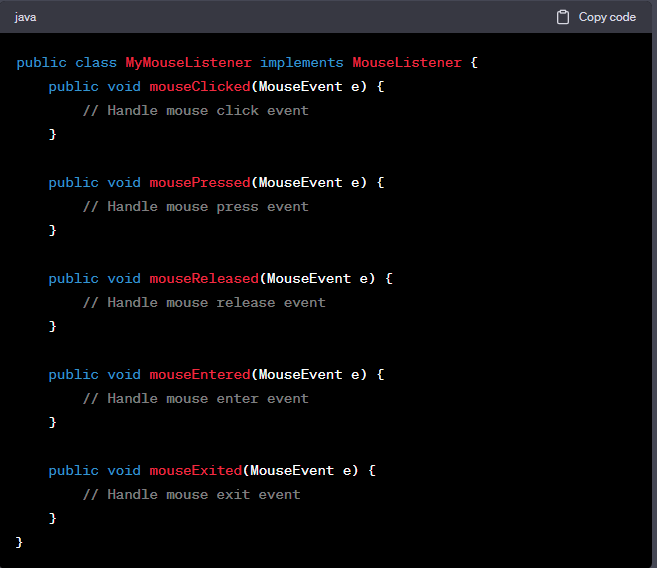
Practical Examples
Let’s delve into practical scenarios to see how MouseListener works in action:
Example 1: Creating a Simple Button
Consider a button that changes color when clicked:
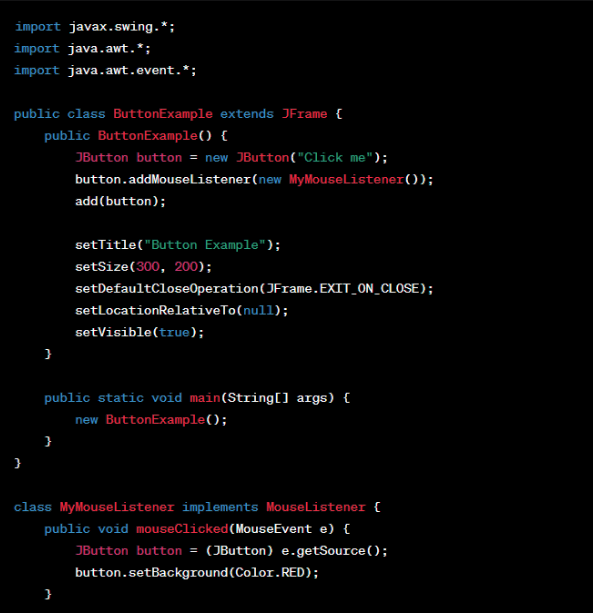
Example 2: Interactive Drawing Panel
Create a drawing panel that allows users to draw when the mouse is pressed:
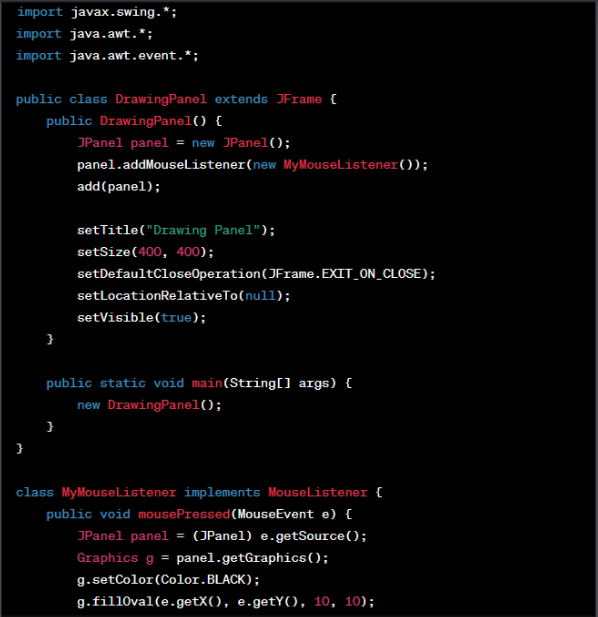
Handling Mouse Motion Events
While MouseListener is perfect for capturing mouse clicks and basic interactions, there are situations where you need to track mouse movement more precisely. This is where MouseMotionListener comes into play.
What is MouseMotionListener?
MouseMotionListener, also a part of Java’s java.awt.event package, provides methods to handle mouse motion events. These events include moving the mouse within a component, dragging it, and releasing it after dragging.
Implementing MouseMotionListener
To implement MouseMotionListener, follow similar steps to implementing MouseListener:
Step 1: Import Required Packages

Step 2: Implement the MouseMotionListener Interface
Create a class that implements the MouseMotionListener interface:
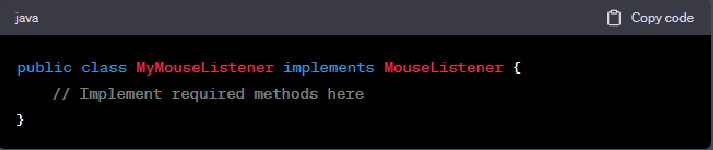
Step 3: Override MouseMotionListener Methods
In your class, override the methods from the MouseMotionListener interface:
- mouseMoved(MouseEvent e): Triggered when the mouse is moved within a component without any buttons being pressed;
- mouseDragged(MouseEvent e): Invoked when the mouse is dragged (moved with a button pressed).
Here’s an example of the implementation:
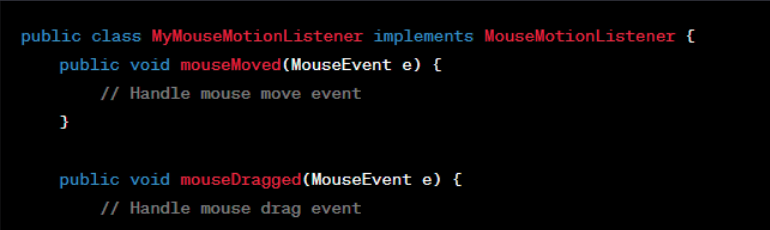
Combining MouseListener and MouseMotionListener
In many cases, you might want to capture both mouse clicks and mouse movement within a single component. To achieve this, you can combine MouseListener and MouseMotionListener interfaces.
Creating a Combined Listener
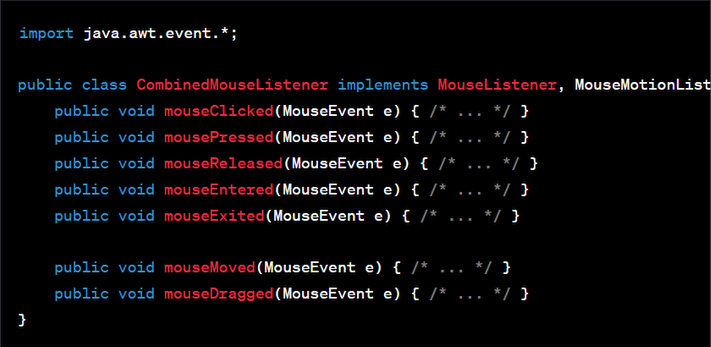
By implementing both interfaces, you can handle a broader range of mouse-related interactions in your application.
Common MouseAdapter Usage
While creating separate classes for MouseListener and MouseMotionListener is effective, Java provides a more concise way using the MouseAdapter class. This class offers default empty implementations for all methods in both interfaces, allowing you to selectively override the ones you need.
Example Usage of MouseAdapter
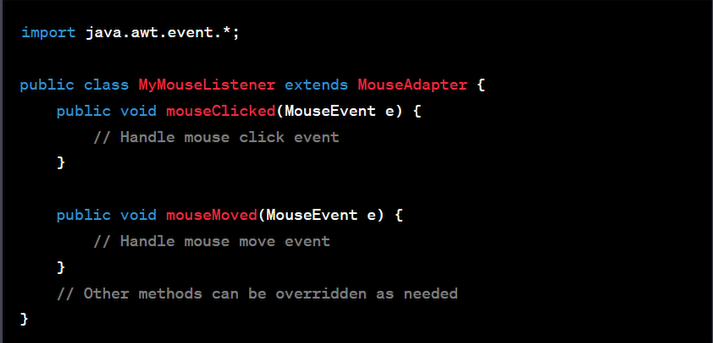
Best Practices for MouseListener Implementation
When implementing MouseListener, consider these best practices:
- Use Appropriate Components: Attach MouseListener to components like buttons, panels, or canvases based on your application’s needs;
- Handle Events Efficiently: Avoid long-running tasks within listener methods to ensure a responsive UI;
- Dispose Resources: If you create resources (like Graphics objects), dispose of them to prevent memory leaks;
- Focus on User Experience: Implement intuitive and meaningful interactions to enhance user experience.
Conclusion
Implementing MouseListener in Java opens the door to interactive and engaging user experiences. You’ve learned the basics, steps, and practical examples of integrating MouseListener into your applications. From simple buttons to interactive drawing panels, you now have the tools to create dynamic interfaces that respond seamlessly to mouse events.
FAQs
MouseListener is used to handle various mouse events like clicking, pressing, releasing, entering, and exiting mouse components in Java applications.
Yes, you can attach MouseListener to components like buttons, panels, and canvases to capture mouse events effectively.
Absolutely! MouseListener is essential for game development as it enables you to capture player interactions and trigger corresponding actions.
Yes, you can change the appearance of components using methods like setBackground(), setForeground(), or repaint() within MouseListener methods.
Yes, Java also provides MouseAdapter, which is an abstract class that implements MouseListener with default empty implementations. You can extend MouseAdapter to override only the methods you need.