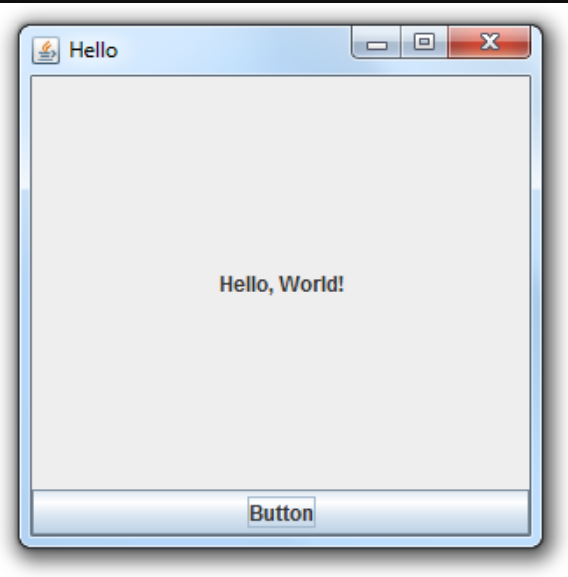
How to Create JButton in Java: Step-by-Step Guide
Buttons are a crucial component of graphic user interfaces (GUI), offering users the ability to initiate actions or navigate through an application. In Java, the JButton class from the Swing framework allows developers to easily incorporate buttons into their applications, making them more user-friendly and interactive.
Let’s explore the process of creating and configuring JButton objects to add both functionality and aesthetics to your Java applications.
Creating a JButton
To start, it’s essential to have a basic understanding of Java programming and Swing components. If you’re new to Java GUI programming, don’t worry – creating a JButton isn’t challenging.
First, import the necessary packages:
- import javax.swing.JButton;
- import javax.swing.JFrame;
- import javax.swing.JPanel.
Next, we’ll create a basic JFrame to place the button:
public class JButtonExample {
public static void main(String[] args) {
JFrame frame = new JFrame("JButton Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(300, 200);
JPanel panel = new JPanel();
frame.add(panel);
JButton button = new JButton("Click Me");
panel.add(button);
frame.setVisible(true);
}
}
In the example above, we’ve created a JFrame named “frame” to house the GUI components. Inside the frame, we’ve added a JPanel named “panel” for layout organization. Within the panel, we’ve placed a JButton named “button” with the label “Click Me”.
Customizing JButton Appearance
While a standard button might suffice, customizing its appearance can make your application visually appealing and engaging. You can modify various JButton aspects, such as its text, icon, background color, and borders.
Text and Icon: Set the button’s text and icon using the setText() and setIcon() methods, respectively. For example:
button.setText("Press Me");
button.setIcon(new ImageIcon("icon.png"));
Background Color: Change the button’s background color with the setBackground() method:
button.setBackground(Color.BLUE);
Borders: Modify the button’s border using the setBorder() method. You can opt for different types of borders like etched, raised, lowered, etc.:
button.setBorder(BorderFactory.createEtchedBorder());
Adding ActionListener for Interaction
Buttons become more appealing when they’re not just decorative but also functional. This can be achieved by attaching an action listener (ActionListener) to the JButton. An ActionListener listens for button presses and responds by performing a predefined action.
Here’s how to implement this:
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
// Execute desired action here
System.out.println("Button pressed!");
}
});
Within the actionPerformed method, you can insert code that should run when the button is pressed. This could be anything from displaying a message, opening a new window, or performing a specific calculation.
Mastering Layout Management in Java GUI Programming
When creating graphical user interfaces (GUI) in the Java programming language, proper component arrangement and organization are crucial for crafting a polished and user-friendly interface. Java offers a variety of layout managers that assist developers in creating flexible and responsive interfaces that adapt to different screen sizes and orientations. In this article, we will delve into the concept of layout management in Java graphical interface programming and explore how to utilize various layout managers to achieve the desired interface design.
The Significance of Layout Management
Layout management refers to the process of defining the position and size of graphical interface components within a container, such as a panel or frame. Effective layout management ensures that the graphical interface remains consistent and functional across different devices and screen sizes.
Java provides several layout managers, each catering to specific design needs and goals.
Types of Layout Managers:
- FlowLayout: This is the default layout manager for most containers in Java. It arranges components in a single row or column, moving to the next row or column when the current one is filled. It is suitable for simple layouts where components do not require precise positioning;
- BorderLayout: BorderLayout divides a container into five regions: North, South, East, West, and Center. Components can be placed in these regions, and they will adjust their sizes based on the container dimensions. This is convenient for creating interfaces with distinct sections;
- GridLayout: GridLayout divides a container into a grid of rows and columns. Each grid cell can hold a component. This layout is ideal for evenly distributing components and is commonly used for forms and tables;
- GridBagLayout: GridBagLayout is a powerful yet complex layout manager that allows for intricate designs. It utilizes a grid, similar to GridLayout, but allows for different weights to be assigned to rows and columns, enabling proportional expansion of components;
- BoxLayout: In BoxLayout, components are arranged in a single line either horizontally or vertically. It is versatile and allows for alignment and size adjustments. To create more intricate layouts, you can nest multiple boxes.
Creating a JButton in Java Example
package com.javapointers.javase;
import java.awt.FlowLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
public class JButtonTest implements ActionListener {
JFrame frame;
JButton button;
public JButtonTest() {
frame = new JFrame();
frame.setTitle("My JFrame");
frame.setSize(500, 400);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
button = new JButton("Click Me!");
button.addActionListener(this);
frame.setLayout(new FlowLayout());
frame.add(button);
frame.setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
System.out.println("You have clicked the button");
}
public static void main(String args[]) {
JButtonTest test = new JButtonTest();
}
}
Conclusions
Adding interactive elements, such as buttons, to Java applications is a fundamental step towards creating user-friendly and engaging software. With the help of the JButton class from the Swing framework, you can easily integrate buttons and customize their appearance to match the application’s style. Attaching ActionListener objects to buttons enables them to trigger specific actions, making the application dynamic and responsive.
As you delve deeper into Java graphical interface programming, explore various layout managers for effective component arrangement and the creation of visually appealing interfaces. Armed with these tools, you’ll be able to develop complex Java applications that offer user convenience.