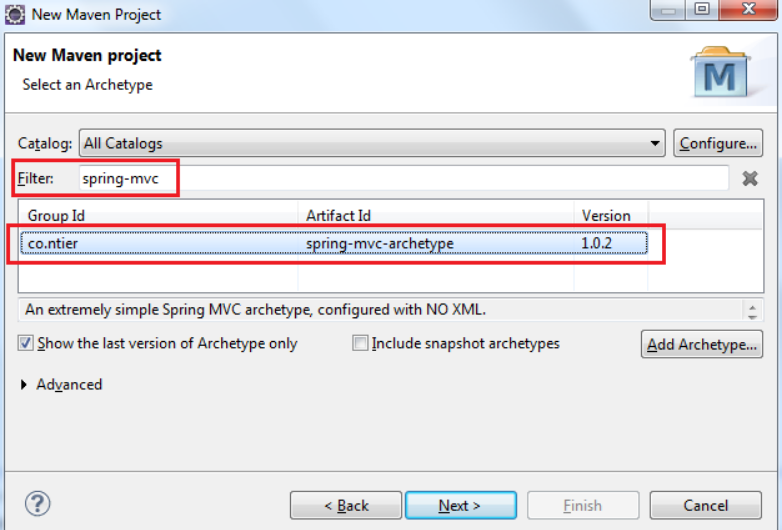
Creating Spring MVC Project Using Maven
In the world of Java web development, Spring MVC has become a reliable framework for creating dynamic and scalable web applications. When combined with Apache Maven, a powerful project management and build automation tool, this process becomes even more organized and efficient.
In this guide, we will walk through the steps of setting up Spring MVC with Maven, allowing you to confidently delve into web development.
Prerequisites
Before you start creating a Spring MVC project using Maven, make sure you have all the necessary tools and components in place. These prerequisites will lay the foundation for smooth and productive development.
Here’s what you need before you begin:
- Java Development Kit (JDK): Ensure that your system has a compatible version of the Java Development Kit (JDK) installed. Spring MVC is built on Java, and having the right JDK version is crucial for compatibility. You can download JDK from the official Oracle website or opt for an open-source alternative like OpenJDK;
- Integrated Development Environment (IDE): Choose an Integrated Development Environment (IDE) that suits your preferences and needs. Popular options for Java development include Eclipse, IntelliJ IDEA, and Visual Studio Code with corresponding Java extensions. An IDE provides a set of tools and features that simplify coding, debugging, and project management;
- Apache Maven: Apache Maven is a powerful tool for project management and build automation. It helps manage dependencies, compile source code, and package applications. Download and install the latest version of Maven from the official Apache Maven website. Maven installation is necessary for configuring and managing the Spring MVC project;
- Basic Java Knowledge: Familiarity with the basics of Java programming is essential. Understanding concepts such as classes, objects, methods, and annotations will greatly assist you when working with the Spring MVC framework and Maven configurations.
By fulfilling these prerequisites, you’ll be well-prepared to create a Spring MVC project using Maven. These tools will form the basis of your development environment, enabling you to efficiently create, test, and deploy web applications. As you progress through the stages of creation and configuration, your understanding of Java, Spring MVC, and Maven will grow, providing a solid foundation for developing dynamic and feature-rich web applications.
Step 1: Creating a Maven Project for Your Spring MVC Application
Creating a Maven project is a fundamental step in setting up a Spring MVC application. Maven simplifies subsequent management, dependency handling, and build processes, providing a structured environment for efficient development. Here’s how to create a Maven project for a Spring MVC application:
- Launch your Integrated Development Environment (IDE). Open your chosen IDE, such as Eclipse, IntelliJ IDEA, or Visual Studio Code, and ensure that it’s properly configured for Java and Maven work;
- Create a new Maven project. In your IDE, navigate to the “File” menu and select “New Project” or a similar option. Choose “Maven” as the project type. This will initiate the project creation wizard;
- Select a template. In the project creation wizard, you’ll be prompted to choose a project template or archetype. Since we want to create a basic Maven project, select the option like “Create a simple project (skip archetype selection)” or its equivalent, depending on your development environment.
Configure Details: Provide the Necessary Information:
- Group ID: Typically, this is an organization’s identifier in reverse domain name style;
- Artifact ID: The artifact ID is the name of your project;
- Version: Specify the initial project version (e.g., 1.0-SNAPSHOT);
- Package: This is the Java package under which your source code will be organized.
Choose Project Location:
- Select a directory where the project will be created. This directory will serve as the project’s root directory;
- Complete project creation: Review the details to ensure accuracy. Then, click the “Finish” button or its equivalent to create the Maven project structure;
- Explore the project structure. After creating the project, you’ll see the standard Maven project structure. It includes directories like src/main/java for Java source code, src/main/resources for resources, and src/test for testing-related files.;
- The pom.xml file in the project root is the Maven Project Object Model (POM) file, which manages the project’s configuration and dependencies.
Congratulations! You have successfully created a basic Maven project for your Spring MVC application. This structured foundation will enable you to easily integrate Spring MVC and other dependencies, manage resources, and perform efficient builds.
In the following steps, you will proceed with configuring Spring MVC and adding necessary dependencies to your Maven project. This will lay the groundwork for simple and effective development of dynamic web applications.
Step 2: Configure Spring MVC Dependencies
Open the pom.xml file of your project. This file manages the project’s dependencies.
Add the following dependencies to include the required Spring MVC components:
xml
<dependencies>
<!-- Spring MVC -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.3.10.RELEASE</version> <!-- Use the latest version -->.
</dependency>
<!-- Other dependencies -->
</dependencies>
Save the pom.xml file. Maven will automatically download and manage these dependencies.
Step 3: Create a Spring MVC Configuration
In the src/main/java directory, create a new Java class for configuring Spring MVC, for example, MvcConfig.java.
- Annotate the class with @Configuration to indicate that this is a configuration class;
- Implement the WebMvcConfigurer interface to configure Spring MVC behavior;
- Override methods to configure view resolvers, resource handling, and other MVC-specific parameters.
Step 4: Create Controllers
Create a new package, for example, controllers, in the src/main/java directory to store your controller classes.
- Create a new Java class for your controller, such as HomeController.java;
- Annotate the class with @Controller to indicate that this is a Spring MVC controller;
- Define methods in the class to handle various requests and map them to corresponding views.
Step 5: Create Views
Inside the src/main/webapp/WEB-INF/views directory, create JSP files that will serve as views.
- These JSP files will be returned by methods in the controller classes;
- Configure the JSP files using HTML, CSS, and any dynamic data from the controller.
Step 6: Launching the Spring MVC Application
Depending on the IDE you’re using, you can run the Spring MVC application using an embedded server such as Tomcat or Jetty:
- After launching the application, open a web browser and navigate to http://localhost:8080/your-artifact-id/;
- You should see that your Spring MVC application is up and running, with controllers handling requests and displaying views.
Congratulations! You have successfully created a Spring MVC project using Maven. This powerful combination allows you to easily develop reliable and scalable web applications. As you delve deeper into Spring MVC, you can explore additional features such as handling submitted forms, integrating with databases, and implementing security measures.
Creating a Controller – Code Example
package com.javapointers.controllers;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
@Controller
public class HomeController {
@RequestMapping(value="/home", method = RequestMethod.GET)
public String viewHome(){
return "home";
}
}
Conclusions
Creating Spring MVC projects using Maven lays a solid foundation for effective web development in Java. With comprehensive Spring MVC support and the ability to manage dependencies through Maven, developers can focus on building feature-rich applications without worrying about project configuration complexities.
By following the step-by-step guide outlined in this article, you can confidently embark on creating dynamic and responsive web applications.